Directives in AngularJS are JavaScript class which is declared as @directive. These are the Document Object Model (DOM) instruction sets, which decide how logic implementation can be done.
Angular directives can be classified into three types:
- Component Directives: It forms the main class and is declared by @Component. It contains the details on component processing, instantiated and usage at run time.
Example: It contains certain parameters some of them are shown in this example.
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
|
There are three parameters discussed below:
- Selector: Tells the template tag which specifies the beginning and end of the component.
- templateURL: Consists of the template used for the component.
- styleUrls: It is of array type which consists of all the style format files used for the template.
- Structural Directives: Structure directives manipulate the DOM elements. These directives have a * sign before the directive. For example, *ngIf and *ngFor.
Example: Let’s look the implementation of *ng-if-else and *ng-for. Using them, we classify weekdays and weekends.
Component file:
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
Weekdays:Array =[
'Sunday' , 'Monday' , 'Tuesday' ,
'Wednesday' , 'Thursday' , 'Friday' , 'Saturday' ]
}
|
Template file:
< div * ngFor = "let day of Weekdays" >
< ng-container * ngIf =
"(day == 'Saturday' || day == 'Sunday'); else elseTemplate" >
< h1 >{{day}} is a weekend</ h1 >
</ ng-container >
< ng-template #elseTemplate>
< h1 >{{day}} is not a weekend</ h1 >
</ ng-template >
</ div >
|
Output: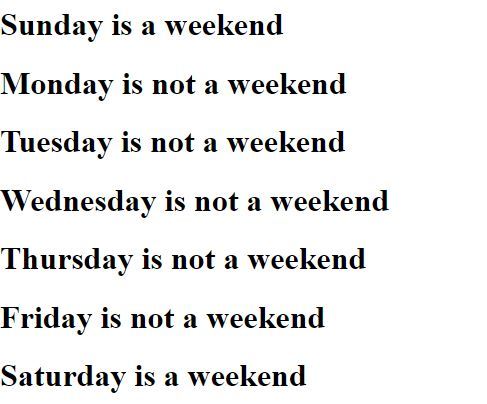
- Attribute Directives: Attribute directives are used to change the look and behavior of the DOM element. It provides the facility to create our own directive.
Example: This example describes how to make our own directive.
Write command as follows:
ng g directive
Directive:
import { Directive, ElementRef, OnInit } from '@angular/core' ;
@Directive({
selector: '[appChanges]'
})
export class ChangesDirective {
constructor(private eltRef: ElementRef) {
this .eltRef.nativeElement.style.backgroundColor = 'green' ;
this .eltRef.nativeElement.style.color = 'white' ;
changing the text color to white
}
ngOnInit() {
}
}
|
The Component File:
import { Component, OnInit, Directive } from '@angular/core' ;
import { ChangesDirective } from '../changes.directive' ;
@Component({
selector: 'app-derived-directive' ,
templateUrl: './derived-directive.component.html' ,
styleUrls: [ './derived-directive.component.css' ]
})
export class DerivedDirectiveComponent implements OnInit {
isClicked:boolean= false ;
constructor() { }
buttonClick(){
this .isClicked = true ;
}
ngOnInit() {
}
}
|
The Template
< button >Click Here</ button >
< div style = "width: 220px;height: 50px" >
< h1 >GeeksForGeeks</ h1 >
</ div >
< div style = "color: green;width: 300px;height: 50px" >
< h1 > GeeksForGeeks</ h1 >
</ div >
|
Output:
- Before clicking the Button:
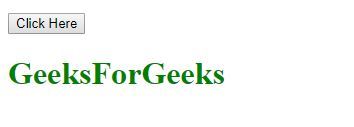
- After clicking the Button:
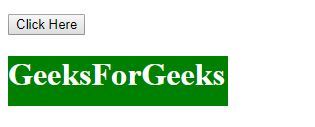
No comments:
Post a Comment